Arrays in C Language
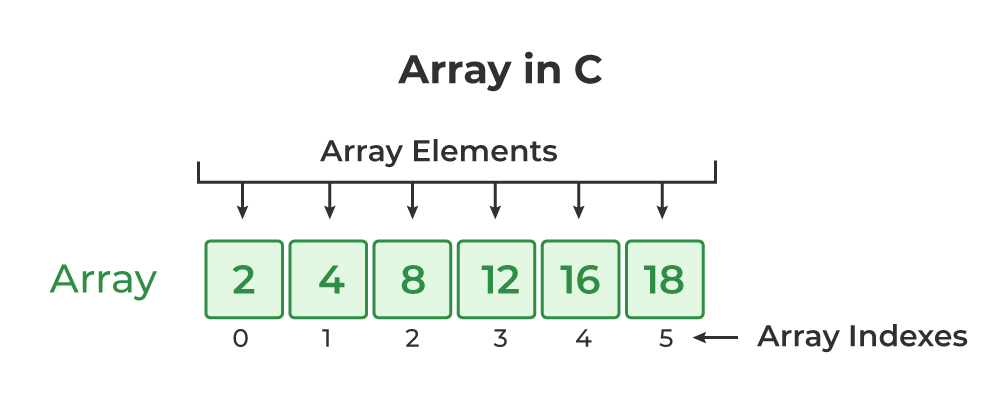
An array is a data structure used in programming to store a collection of elements, typically of the same type, in a fixed-size sequence.
Initialisation of Array
1#include <stdio.h>
2int main()
3{
4 // it's not important to give size during initialisation.
5 // eg: float y[]={1.2,2,3.32};
6
7 int g[5];
8 int i;
9
10 printf("\n enter the elements of array");
11 for (i = 0; i < 5; i++)
12 {
13 scanf("%d", &g[i]);
14 }
15 printf("values of array are \n");
16 for (i = 0; i < 5; i++)
17 {
18 printf("\n value of g[%d]=%d", i, g[i]);
19 }
20 return 0;
21}
To read an Array from keyboard
1#include <stdio.h>
2int main()
3{
4
5 int a[3][3];
6 for (int i = 0;i<3;i++){
7 for(int j = 0;j<3;j++){
8 printf("Enter the value of matrix [%d][%d]: ", i,j);
9 scanf("%d", &a[i][j]);
10 }
11 }
12
13
14
15// print value read by keyboard
16for (int i = 0;i<3;i++){
17 for(int j = 0;j<3;j++){
18 printf("\n print the value of matrix [%d][%d]: %d", i,j, a[i][j]);
19 }
20}
21
22return 0;
23}
Example of char array with initialization
1#include<stdio.h>
2int main(){
3char city[][10] = {"Jaipur","Jhodpur","Jaisalmer"};
4
5for (int i = 0;i<3;i++){
6 for(int j = 0;j<3;j++){
7 printf("\n print the value of matrix [%d][%d]: %s", i,j, city[i][j]);
8 }
9}
10return 0;
11}
To print the characters of an Array
1#include<stdio.h>
2void main{
3char angel[] = "angelvyas";
4printf("the value of char is %c", angel[6]);
5 printf("the value of x is %d", x);
6}
Sum of Arrays
1#include <stdio.h>
2int main()
3{
4 int a[10], n, i, sum = 0;
5 printf("\nenter the size of an array");
6
7 scanf("%d", &n);
8 printf("enter the elements of the array");
9
10 for (i = 0; i < n; i++)
11 {
12 scanf("%d", &a[i]);
13 }
14 printf("\nthe given array is");
15 for (i = 0; i < n; i++)
16 {
17 printf("%d", a[i]);
18 printf("\n");
19 }
20 printf("\nthe sum of elements of the given array is");
21 for (i = 0; i < n; i++)
22 {
23 sum = sum + a[i];
24 }
25 printf("%d", sum);
26 return 0;
27}
2D Array:
1#include <stdio.h>
2
3int main(){
4 int a[4][5];
5 int i,j;
6 printf("enter the value for array: \n");
7 for(i=0;i<4;i++){
8 for(j=0;j<5;j++)
9 scanf("%d", &a[i][j]);
10 }
11 printf("\nthe value of array is");
12 for(i=0;i<4;i++){
13 for(j=0;j<5;j++){
14 printf("value of a[%d][%d]=%d", i,j,a[i][j]);
15 }
16 return 0;
17 }
18}