Basics of C-language
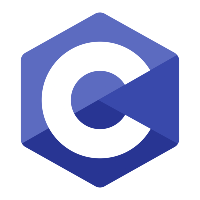
C is a powerful, general-purpose programming language that provides control over system resources and efficient execution. This post will cover some essential concepts in C that every programmer should understand: Control Strings, Global Variables, Strings, Switch Statements, Ternary Operators, Unformatted Input/Output, Bitwise Operators, and Algebraic Operations.
Control String
Control strings are used in functions like printf
and scanf
to format input and output. They consist of placeholders like %d for integers, %f for floating-point numbers, and %s for strings. Here's a simple example:
1#include<stdio.h>
2int main()
3{
4 int x = -23;
5 float y = 2.34;
6 char z[] = "angelvyas";
7 long p = 2222222222;
8 unsigned w = 3.4;
9
10 printf("the value of x is %d", x);
11 printf("\n the vlaue of y is %f", y);
12 printf("\n the value of char is %c", z[4]);
13 printf("\b the value of p is %ld", p);
14 printf ("\t the value of w is %u", w);
15 return 0;
16}
Global Variables
Global variables are declared outside of any function and can be accessed by any function in the program. They retain their value throughout the program's execution. Use global variables sparingly, as they can make debugging difficult if overused.
1#include <stdio.h>
2#define x 20
3
4int main()
5{
6 printf("hi the value of x is- %d", x);
7 return 0;
8}
To print a value
1#include <stdio.h>
2int main()
3{
4 int i=1;
5 while(i<=10)
6 {
7 printf("%d \n", i);
8 i++;
9 }
10 return 0;
11}
String initialisation and printing
1#include <stdio.h>
2#include <string.h>
3int main()
4{
5 //char name[7][6]={"mango","banana", "apple", "orange", "pineapple", "dragonfruit", "litchi"};
6 //printf("%c",name[2][3]);
7
8 char a[2]="ai";
9 printf("%s",a[0]);
10 return 0;
11}
Switch Statement
1#include <stdio.h>
2int main()
3{
4 int a, b;
5 char c,d;
6 printf("enter the value for operators:\n");
7 scanf("%d%d", &a, &b);
8
9 scanf("%c", &c);
10 printf("give the operator:\n");
11 scanf("%c", &c);
12
13 switch (c)
14 {
15 case '+':
16 d = a + b;
17 printf("answer is %d", d);
18 break;
19 case '*':
20 d = a * b;
21 printf("answer is %d", d);
22 break;
23
24 default:
25 printf("no");
26 }
27 return 0;
28}
Turnery Operator
1#include <stdio.h>
2
3int main()
4{
5 int a = 20;
6 int b = (a < 8) ? 0 : 1;//after question mark it is if area anf after colon it is else area
7 printf("the value of b is %d", b);
8 return 0;
9
10}
Unformattedinputoutput
1#include <stdio.h>
2
3int main()
4{
5 // getc: It reads a single character from a given input stream
6 printf("Print the one char: ");
7 printf("%c",getc(stdin));
8
9 // The difference between getc() and getchar() is
10 // getc() can read from any input stream,
11 // but getchar() reads a single input character from standard input.
12 // So getchar() is equivalent to getc(stdin).
13 printf("\n Example of getchar \n");
14 printf("%c", getchar());
15 return 0;
16}
Bitwise Operator
1#include <stdio.h>
2int main()
3{
4 int a=4;
5 int b=5;
6 printf("the value of bitwise and %d", a&b);
7 printf("the value of bitwise or %d", a|b);
8 printf("the value of bitwise not %d", ~a);
9 return 0;
10
11
12}
Scientific Notation Datatype
1#include<stdio.h>
2int main(){/*scientific notation datatype*/
3 double a=56.23;
4 char y='a';
5
6 printf("%e %d",a,y);
7 return 0;
8}
Algebraic Operations
1#include <stdio.h>
2int main()
3{ int a,b;
4 printf("enter the number:");
5 scanf("%d%d", &a,&b);
6 printf("addition is: %d",a+b);
7 printf("subtraction is:%d", a-b);
8 printf("mutiplication is:%d", a * b);
9 printf("modulas is:%d", a % b);
10 0;
11}
To take input operator and operands then perform the operation.
1#include <stdio.h>
2#include <math.h>
3int main()
4{
5 int a, b;
6 char c;
7 printf("enter the numbers:");
8 scanf("%d%d", &a, &b);
9 scanf("%c", &c);
10 printf("enter the operator:");
11 scanf("%c", &c);
12 if (c == '+')
13 {
14 printf("anwer:%d", a + b);
15 }
16 else if (c == '-')
17 {
18 printf("answer:%d", a - b);
19 }
20 else if (c == '*')
21 {
22 printf("answer:%d", a * b);
23 }
24 else
25 {
26 printf("answer:%d", a / b);
27 }
28 return 0;
29}