Loops in C-language
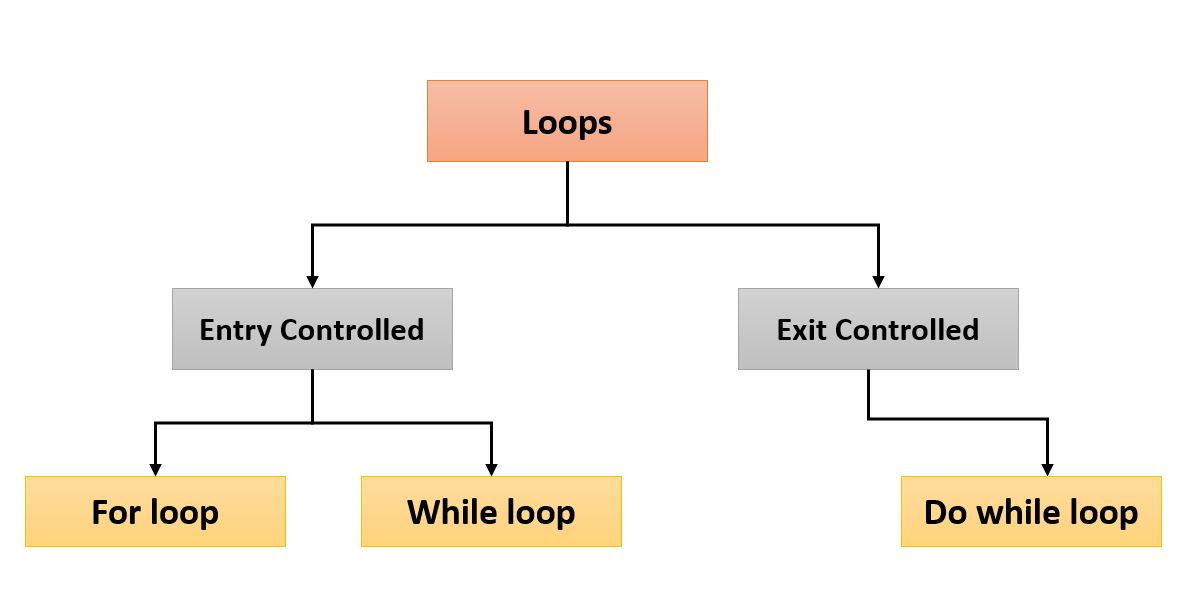
Loops in C language are used to repeatedly execute a block of code based on certain conditions. They are fundamental for tasks such as iterating over arrays, processing data, and performing repetitive operations.
Loops
1#include <stdio.h>
2#include <string.h>
3
4void main()
5{
6 // loop means - to execute the statement while the condition satisfied
7 // for and while are two method mainly used for loops(do while is also there)
8
9 // condition gives output in the form of 1 = True 0 = False
10
11 int i = 0; // assignment
12 while(i <= 10) // condition
13 {
14 printf("Hi this is the example of loop %d \n", i);
15 i = i + 1; // increment
16 }
17
18 while(1) // condition
19 {
20 printf("Hi this is the example of loop %d \n", i);
21 i = i + 1; // increment
22
23 if (i <= 10){
24 continue;
25 }else{
26 break;
27 }
28
29 }
30
31
32 // looping using for loop
33 for(int i = 0; i <= 10; i = i + 1)
34 {
35 printf("Hi all - %d \n", i);
36 }
37
38}
Example Questions-
Pyramids
1
22
333
4444
1#include <stdio.h>
2int main()
3{
4 int n,i,j;
5 printf("enter the value for n\n");
6 scanf("%d", &n);
7 for (i = 1; i <= n; i++)
8 {
9 for (j = 1; j <= i; j++)
10 {
11 printf("%d", i);
12 }
13 printf("\n");
14 }
15 return 0;
16}
12
123
1234
1#include <stdio.h>
2int main()
3{
4 int n,i,j;
5 printf("enter the value for n");
6 scanf("%d", &n);
7 for (i = 1; i <= n; i++)
8 {
9 for (j = 1; j <= i; j++)
10 {
11 printf("%d", j);
12 }
13 printf("\n");
14 }
15 return 0;
16}
Fibonnaci Series
1#include <stdio.h>
2int main()
3{
4 int n,i;
5 printf("enter the value of n:\n");
6 scanf("%d", &n);
7 int t1=0,t2=1,t3=t1+t2;
8 printf("%d\n%d", t1,t2);
9 for(i=3;i<=n;++i){
10 printf("\n%d", t3);
11 t1=t2;
12 t2=t3;
13 t3=t1+t2;
14 }
15 return 0;
16
17}
Geometric Series
1#include <stdio.h>
2int main()
3{
4 int x, n, count, sum, i;
5 printf("enter the value for x and n:\n");
6 scanf("%d%d",&x,&n);
7 i = 1;
8 count = 1;
9 sum = 1;
10 while (i <= n)
11 {
12 count = count * x;
13 sum = sum + count;
14 i++;
15 }
16 printf("sum is %d", sum);
17 return 0;
18}
Factorial
1#include<stdio.h>
2void main()
3{ int fact(int a);
4 int n,z;
5 printf("enter the number:");
6 scanf("%d",&n);
7 z=fact(n);
8 printf("the factorial of the given number is:%d",z);
9}
10int fact(int a)
11{ int i,c=1,res;
12 for(i=1;i<=a;i++)
13 {
14 res=i * c;
15 c=res;
16
17 }
18 return c;
19
20}
Using Reccursion-
1#include <stdio.h>
2int fact(int a);
3void main()
4{
5 int num, res;
6 printf("enter the number:");
7 scanf("%d", &num);
8 res = fact(num);
9 printf("result is %d", res);
10}
11int fact(int n)
12{
13 if (n == 1)
14 {
15 return 1;
16 }
17 else
18 {
19
20 return (n * fact(n - 1));
21 }
22}
GCD
1#include<stdio.h>
2int main()
3{
4 int a,b,c,temp;
5 int gcd(int m,int n);
6 printf("enter the values for a and b.\n");
7 scanf("%d%d",&a,&b);
8 if(a<b){
9 temp=a;
10 a=b;
11 b=temp;
12
13 }
14 c=gcd(a,b);
15 printf("gcd of given two numbers is:%d",c);
16 return 0;
17}
18int gcd(int m,int n){
19 int rem;
20 rem=m%n;
21 while(rem!=0){
22 m=n;
23 n=rem;
24 rem=m%n;
25
26 }
27 return n;
28}
Using Reccursion-
1#include <stdio.h>
2int gcd(int m, int n);
3void main()
4{
5 int x, y, z;
6 printf("enter two numbers \n");
7 scanf("%d%d", &x, &y);
8 z = gcd(x, y);
9 printf("gcd of two number is %d", z);
10}
11int gcd(int m, int n)
12{
13 if (n != 0)
14 {
15 return gcd(n, m % n);
16 }
17 else
18 {
19 return m;
20 }
21}