Searching in C-language
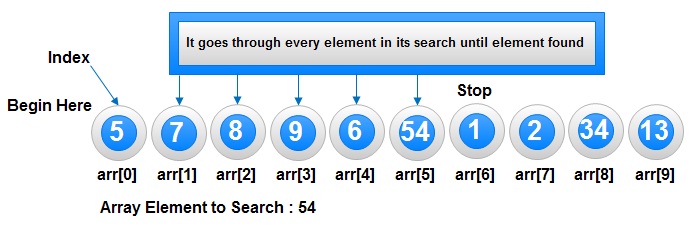
Searching in C language refers to the process of finding a specific element or value within a data structure, typically an array. Searching algorithms help determine whether an element exists in the data structure and, if so, its position.
Searching:
L-Search
1#include<stdio.h>
2int lsearch(int b[],int n,int key);
3void main()
4{
5 int a[20],num,k,loc,i;
6 printf("\nEnter the number of elements in the list:");
7 scanf("%d",&num);
8 printf("Enter the elements of the list:\n");
9 for(i=0;i<num;i++)
10 scanf("%d",&a[i]);
11 printf("Enter the key you are searching for:\n");
12 scanf("%d",&k);
13 loc = lsearch(a,num,k);
14 if(loc!=-1)
15 printf("successful search %d is found at %d\n",k,loc+1);
16 else{
17 printf("unsuccessful search\n");
18 }
19
20}
21int lsearch(int b[],int n,int key)
22{
23 int i;
24 for(i=0;i<n;i++)
25 {
26 if(b[i]==key)
27 return i;
28 }
29 return -1;
30}
B-Search
1#include <stdio.h>
2int bsearch(int a[], int n, int key);
3void main()
4{
5 int a[20], n, i, k, pos = -1;
6 printf("Enter the number of elements in the given array:\n");
7 scanf("%d", &n);
8 printf("Enter the elements of an array:\n");
9 for (i = 0; i < n; i++)
10 {
11 scanf("%d", &a[i]);
12 }
13 printf("Enter the key you are searching for:\n");
14 scanf("%d", &k);
15 pos = bsearch(a, n, k);
16 if (pos != -1)
17 {
18 printf("the key %d is found at %d", k, pos + 1);
19 }
20 else
21 printf("the key is not found\n");
22}
23int bsearch(int a[], int n, int key)
24{
25 int first = 0, last = n - 1, middle;
26 while (first <= last)
27 {
28 middle = (first + last) / 2;
29 if (key < a[middle])
30 last = middle - 1;
31 else if (key > a[middle])
32 first = middle + 1;
33 else
34 return middle;
35 }
36}