Sorting in C-language
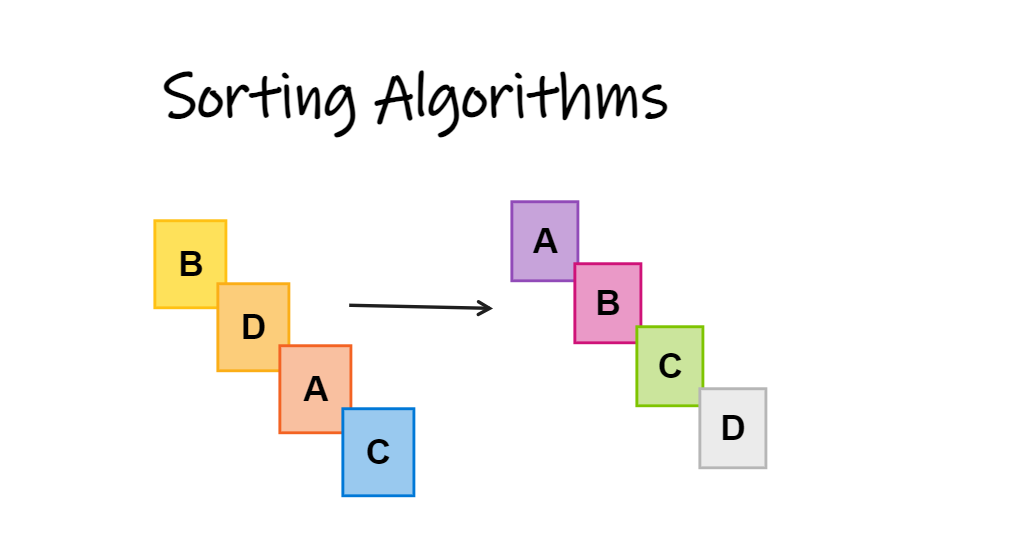
Sorting in C language refers to arranging the elements of an array in a specific order, typically in ascending or descending order.
Sorting:
Bubble Sort
1#include <stdio.h>
2int main()
3{
4 int i, j, k, temp, n, a[20];
5
6 printf("enter the number of elements in the array:\n");
7 scanf("%d", &n);
8 printf("\nenter the elements of the array:");
9 for (i = 0; i < n; i++)
10 {
11 scanf("%d", &a[i]);
12 }
13 for (i = 1; i < n; i++)
14 {
15 for (j = 0; j < n - 1; j++)
16 {
17 if (a[j] > a[j + 1])
18 {
19 temp = a[j];
20 a[j] = a[j + 1];
21 a[j + 1] = temp;
22 }
23 }
24
25 printf("pass %d\n", i);
26
27 for (k = 0; k < n; k++)
28 {
29 printf("%d", a[k]);
30 }
31 printf("\n");
32
33 }}
Insertion Sort
1 #include <stdio.h>
2int main()
3{
4 int i, j, n, temp, a[30];
5 printf("enter the number of elements in the array:\n");
6 scanf("%d", &n);
7 printf("\nenter the elements of the array");
8 for (i = 0; i < n; i++)
9 {
10 scanf("%d", &a[i]);
11 }
12
13 for (i = 1; i < n; i++)
14 {
15
16 j = i;
17 while ((a[j-1]>a[j]) && (j >= 0))
18 {
19
20 temp=a[j];
21 a[j]=a[j-1];
22 a[j-1]=temp;
23 j--;
24 }
25
26
27 }
28 printf("sorted list is\n");
29
30 for (i = 0; i < n; i++)
31 {
32 printf("%d\n", a[i]);
33 }
34
35 return 0;
36}
Selection Sort
1#include <stdio.h>
2
3void swap(int *xp, int *yp)
4{
5 int temp = *xp;
6 *xp = *yp;
7 *yp = temp;
8}
9
10void selection(int a[], int n)
11{
12 int i, j, min;
13 for (i = 0; i < n; i++)
14 {
15 min = j;
16 printf("a[%d]:%d\n", i, a[i] );
17 for (j = i + 1; j < n; j++)
18 {
19 printf("a[%d]:%d ", j, a[j] );
20 if (a[j] < a[i])
21 {
22 swap(&a[i], &a[j]);
23 }
24 }printf("\n");
25 }
26}
27void www(int a[], int size)
28{
29 int i;
30 for (i = 0; i < size; i++)
31 {
32 printf("%d\n", a[i]);
33 }
34 printf("\n");
35}
36
37int main()
38{
39 int a[] = {5,6,4,3,2};
40 int n = 5;
41 selection(a, n);
42 printf("sorted array:\n");
43 www(a, n);
44 return 0;
45}