DS - Simple Queue
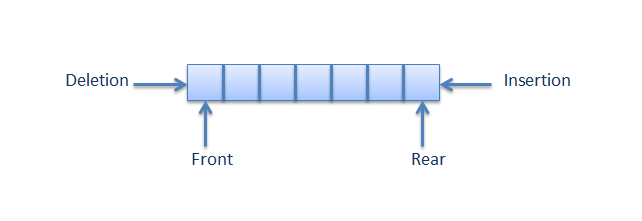
A simple queue
is a fundamental data structure that follows the First-In, First-Out (FIFO)
principle. This means that the first element added to the queue will be the first one to be removed, just like people standing in line—whoever gets in first is served first.
Key Operations in a Simple Queue:
Enqueue (Insertion)
: Adding an element to the back of the queue.Dequeue (Removal)
: Removing an element from the front of the queue.Peek (Front)
: Viewing the element at the front of the queue without removing it.isEmpty
: Checking if the queue is empty.
Example: Think of a line at a movie theater. The first person in line buys the ticket first, and as more people arrive, they join at the back of the line. When a person buys a ticket and leaves, the next person in line gets their turn.
Visual Example:
Initial queue: [1, 2, 3] (1 is at the front, 3 is at the back)Enqueue(4): [1, 2, 3, 4]Dequeue(): [2, 3, 4] (1 is removed)
Applications:
Task scheduling
(e.g., in operating systems)Breadth-First Search (BFS)
in graph traversalManaging resources
in simulations
It’s a simple, efficient way to handle processes where order matters!
Programs:
Implementation of Simple Queue.
1#include <stdio.h>
2#include <stdlib.h>
3#define n 5
4int a[n];
5int f = -1;
6int r = -1;
7void enqueue(int e)
8{
9 if (r == n - 1)
10 {
11 printf("Q is full\n");
12 }
13 else
14 {
15 if (f == -1 && r == -1)
16 {
17 f = 0;
18 r = 0;
19 }
20 else
21 {
22 r++;
23 }
24 a[r] = e;
25 printf("inserted successfully\n");
26
27 }
28}
29
30void dequeue()
31{
32 if (f == -1 && r == -1)
33 {
34 printf("Q is empty\n");
35 }
36 else
37 {
38 if (f == r)
39 {
40 f = -1;
41 r = -1;
42 }
43 else
44 {
45 f++;
46 }
47 }
48}
49
50void display()
51{
52 if (f == -1 && r == -1)
53 {
54 printf("queue is empty\n");
55 }
56 else
57 {
58 printf("Queue:\n");
59 for (int i = f; i <= r; i++)
60 {
61 printf("%d\t", a[i]);
62 }
63 printf("\n");
64 }
65}
66
67int main()
68{
69 int ch, d, c;
70 while (1)
71 {
72 printf("1. enqueue operation\n");
73 printf("2. dequeue operation\n");
74 printf("3. Display queue\n");
75 printf("4. exit\n");
76 printf("enter your choice\n");
77 scanf("%d", &ch);
78 switch (ch)
79 {
80 case 1:
81 printf("enter the data\n");
82 scanf("%d", &d);
83 enqueue(d);
84 break;
85 case 2:
86 dequeue();
87 break;
88
89 case 3:
90 display();
91 break;
92
93 case 4:
94 exit(0);
95 break;
96
97 default:
98 printf("invalid operation\n");
99 }
100 }
101}
Queue using Linked List.
1#include <stdio.h>//q using linked list
2#include <stdlib.h>
3
4struct node
5{
6 struct node *next;
7 int data;
8};
9
10struct node *f, *r;
11
12void enqueue()
13{
14 int a;
15 printf("\nEnter the data value\n");
16 scanf("%d", &a);
17
18 struct node *nn = (struct node *)malloc(sizeof(struct node));
19 nn->data = a;
20 nn->next = NULL;
21
22 if (r == NULL)
23 {
24 f = nn;
25 r = nn;
26 }
27
28 else
29 {
30
31 r->next = nn;
32 r = nn;
33 }
34}
35
36void display()
37{
38 struct node *temp;
39 temp = f;
40 printf("\nThe Queue list is as:");
41
42 while (temp->next != NULL)
43 {
44 printf("\n%d", temp->data);
45 temp = temp->next;
46 }
47
48 printf("\n%d", temp->data);
49}
50
51void dequeue()
52{
53 if (f != NULL)
54 f = f->next;
55}
56
57void main()
58{
59
60 while (1)
61 {
62 int ch;
63
64 printf("\nMENU\n");
65 printf("1.enqueue\n");
66 printf("2.display\n");
67 printf("3.dequeue\n");
68 printf("4.exit\n");
69 printf("Enter choice:\n");
70 scanf("%d", &ch);
71
72 switch (ch)
73 {
74
75 case 1:
76 enqueue();
77 break;
78 case 2:
79 display();
80 break;
81 case 3:
82 dequeue();
83 break;
84 case 4:
85 exit(0);
86
87 default:
88 printf("Invalid\n");
89 }
90 }
91}