DS - Stack
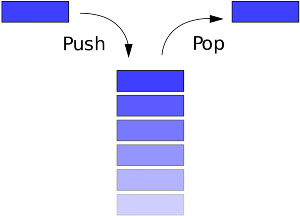
A stack is a fundamental data structure in computer science that operates on a Last In, First Out (LIFO) principle. This means that the last element added to the stack is the first one to be removed. You can think of a stack like a stack of plates: you add new plates to the top and remove them from the top as well.
Key Operations
Push
: Add an element to the top of the stack.Pop
: Remove the element from the top of the stack.Peek (or Top)
: Retrieve the element at the top of the stack without removing it.IsEmpty
: Check if the stack is empty.
Characteristics
LIFO Order
: The most recently added element is the first one to be removed.Dynamic Size
: In many implementations, a stack can grow or shrink as needed, depending on the underlying data structure (such as an array or linked list).
Program
1#include <stdio.h>
2
3#include <stdlib.h>
4
5#define SIZE 4
6
7int top = -1, inp_array[SIZE];
8void push();
9void pop();
10void show();
11
12int main()
13{
14 int choice;
15
16 while (1)
17 {
18 printf("\nPerform operations on the stack:");
19 printf("\n1.Push the element\n2.Pop the element\n3.Show\n4.End");
20 printf("\n\nEnter the choice: ");
21 scanf("%d", &choice);
22
23 switch (choice)
24 {
25 case 1:
26 push();
27 break;
28 case 2:
29 pop();
30 break;
31 case 3:
32 show();
33 break;
34 case 4:
35 exit(0);
36
37 default:
38 printf("\nInvalid choice!!");
39 }
40 }
41}
42
43void push()
44{
45 int x;
46
47 if (top == SIZE - 1)
48 {
49 printf("\nOverflow!!");
50 }
51 else
52 {
53 printf("\nEnter the element to be added onto the stack: ");
54 scanf("%d", &x);
55 top = top + 1;
56 inp_array[top] = x;
57 }
58}
59
60void pop()
61{
62 if (top == -1)
63 {
64 printf("\nUnderflow!!");
65 }
66 else
67 {
68 printf("\nPopped element: %d", inp_array[top]);
69 top = top - 1;
70 }
71}
72
73void show()
74{
75 if (top == -1)
76 {
77 printf("\nUnderflow!!");
78 }
79 else
80 {
81 printf("\nElements present in the stack: \n");
82 for (int i = top; i >= 0; --i)
83 printf("%d\n", inp_array[i]);
84 }
85}