Multithreading in Java
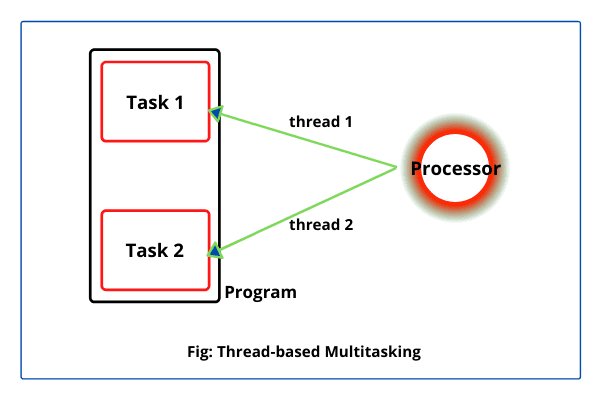
Multithreading
in Java is a powerful feature that allows concurrent execution of two or more threads within a program. A thread is a lightweight subprocess and the smallest unit of processing, and multithreading helps improve the performance and efficiency of applications, especially those requiring multiple simultaneous operations.
Key Concepts of Multithreading in Java:
Thread
: A thread is a separate path of execution in a program. Java supports threads through the java.lang.Thread class and the java.lang.Runnable interface.
Concurrency
: Multithreading enables Java programs to perform multiple tasks simultaneously, making better use of CPU resources. This is particularly beneficial in applications that involve heavy computations or multiple independent operations.
Lifecycle of a Thread:
New
: The thread is created but not yet started.
Runnable
: The thread is ready to run and waiting for CPU allocation.
Running
: The thread is executing its task.
Blocked/Waiting
: The thread is temporarily inactive, waiting for resources or other threads.
Terminated
: The thread has completed its execution.
Creating Threads
Threads in Java can be created in two ways:
- By
extending
theThread class
. - or by
implementing
theRunnable Interface
.
1class Mythread extends Thread {
2 public void run() {
3 for (int i = 10; i >= 0; i--) {
4 System.out.println("Thread #1:" + i);
5 try {
6 Thread.sleep(1000);
7 } catch (InterruptedException e) {
8 e.printStackTrace();
9 }
10
11 }
12 System.out.println("Thread #1 is finished:");
13
14 }
15
16 public static void main(String args[]) throws InterruptedException {
17 Mythread thread1 = new Mythread();
18 Myrunnable runnable1 = new Myrunnable();
19 Thread thread2 = new Thread(runnable1);// in this we're passing the object of our class Myrunnabe as the thread
20 // will start running the object which is passed into it will also start
21 // running, like a cart connected to a horse.
22 thread1.start();
23 thread1.join();// if we put the value of seconds then for 3seconds thread1 will run first.(CPU utilization)
24 thread2.start();
25 System.out.println(1 / 0);// there is an exception still the code is running because threads are
26 // independent of each other main thread is different, thread1 is
27 // different,thread2 is defferent.
28 }
29}
30
31class Myrunnable implements Runnable {// when we are implementing any class it must override all methods otherwise the
32 // class will be declared abstract, Runnable class has only run method.
33 public void run() {
34
35 for (int i = 0; i <= 10; i++) {
36 System.out.println("Thread #2:" + i);
37 try {
38 Thread.sleep(1000);
39 } catch (InterruptedException e) {
40 e.printStackTrace();
41 }
42
43 }
44 System.out.println("Thread #2 is finished:");
45
46 }
47}
Some important useful methods in Java
1class Main {
2 public static void main(String[] args) throws InterruptedException {// this will throw the exception which might
3 // occur when the thread is in sleep mode while
4 // in for loop.
5 System.out.println(Thread.activeCount());
6 Thread.currentThread().setName("MAINNN");
7 System.out.println(Thread.currentThread().getName());
8 Thread.currentThread().setPriority(3);
9 System.out.println(Thread.currentThread().getPriority());
10 System.out.println(Thread.currentThread().isAlive());
11 for (int i = 3; i >= 0; i--) {
12 System.out.println(i);
13 System.out.println();
14 Thread.sleep(1000);
15 }
16
17 Mythread thread2 = new Mythread();
18 thread2.setDaemon(true);
19 System.out.println(thread2.isDaemon());
20 thread2.start();// this start functions executes our run function without telling it to execute.
21 System.out.println(thread2.isAlive());
22
23 }
24}
25
26class Mythread extends Thread {
27 public void run() {
28 System.out.println("This thread is running");
29
30 }
31}